This tutorial will teach you on how to send email/verification email in PHP/MySQLi using mail() function. The function will both work in local server(ex. XAMMP) or hosted server(online) but in case you wanted to send email via the local server, you need to modify your local server to allow it to send email. In my case, I'm using XAMMP, so, I'm going to show you how to set up your XAMMP to send email.
Setting up Local Server to Send Email
Configure php.ini
1. Open php.ini in C:\xampp\php\php.ini.
2. Find extension=php_openssl.dll and remove the semicolon from the beginning of that line.
3. Find [mail function], enable the ff. by removing the semicolon at the start of the line then, change their values:
2. Find extension=php_openssl.dll and remove the semicolon from the beginning of that line.
3. Find [mail function], enable the ff. by removing the semicolon at the start of the line then, change their values:
- SMTP=smtp.gmail.com
- smtp_port=465
- sendmail_from = ndevierte@gmail.com
- sendmail_path = "C:\xampp\sendmail\sendmail.exe -t"
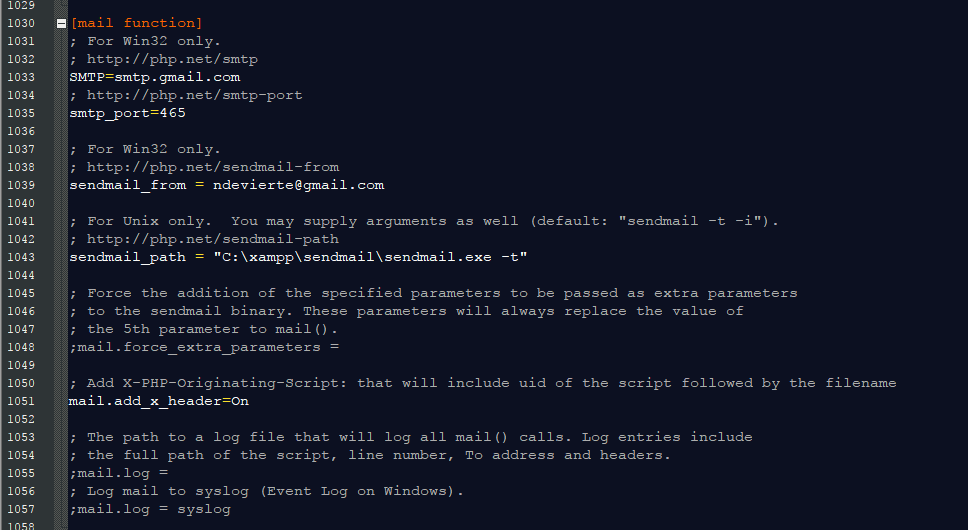
1. Open sendmail.ini in C:\xampp\sendmail\sendmail.ini
2. Find [sendmail], enable the ff. by removing the semicolon at the start of the line, then change their values:
2. Find [sendmail], enable the ff. by removing the semicolon at the start of the line, then change their values:
- smtp_server=smtp.gmail.com
- smtp_port=465
- auth_username=ndevierte@gmail.com
- auth_password=yourpassword
- force_sender=ndevierte@gmail.com
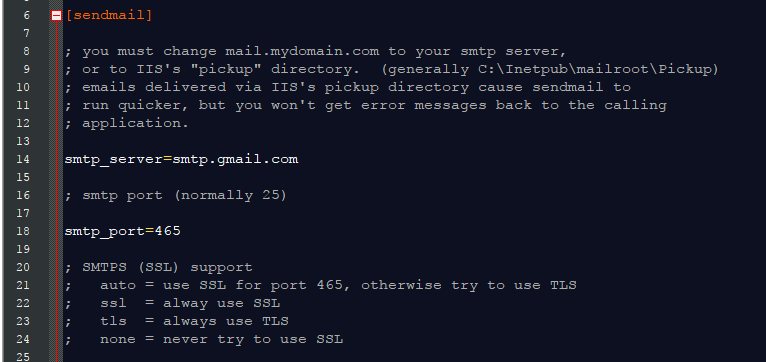
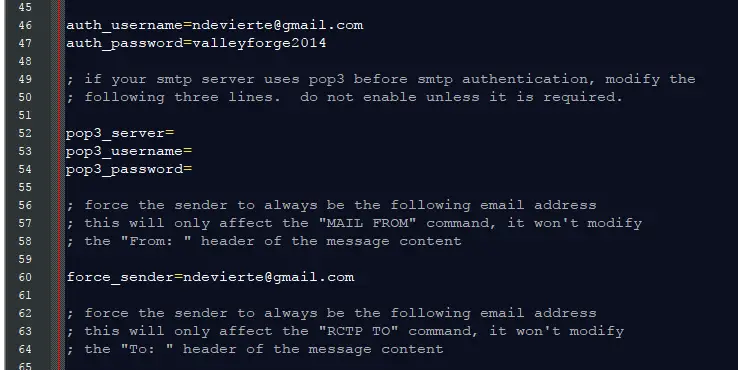
Lastly. make sure to turn this on to allow XAMMP to send email to your email. Use this link.
And you're done. Restart XAMMP and you should now be able to send email using your XAMMP.
Working Example
To test if your XAMMP is working, here's our example. We will create a registration with verification email.
NOTE: Scripts and CSS used in this tutorial are hosted, so, you might need internet connection for them to work.
Creating our Database
First step is to create our database.
1. Open phpMyAdmin.
2. Click databases, create a database and name it as sample.
3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction.
1. Open phpMyAdmin.
2. Click databases, create a database and name it as sample.
3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction.
CREATE TABLE `user` ( `userid` INT(11) NOT NULL AUTO_INCREMENT, `email` VARCHAR(50) NOT NULL, `password` VARCHAR(50) NOT NULL, `code` VARCHAR(20) NOT NULL, `verify` INT(1) NOT NULL, PRIMARY KEY(`userid`) ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
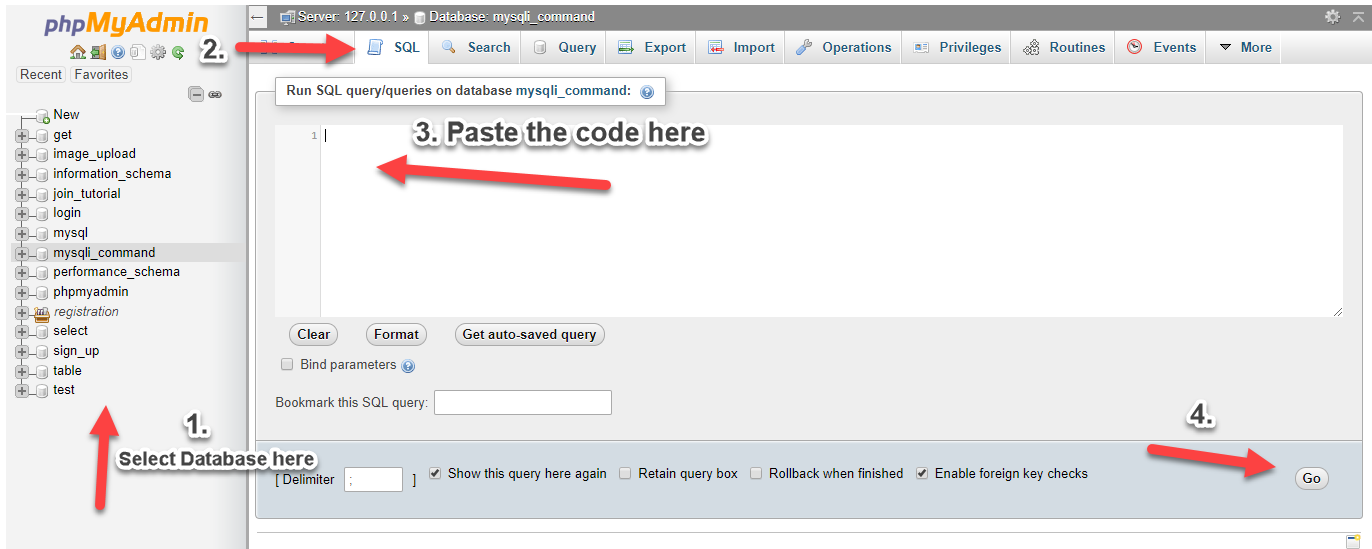
Creating our Connection
Next, we create our connection to our database. This will serve as the bridge between our forms and database. We name this as conn.php.
<?php $conn = mysqli_connect("localhost","root","","sample"); if (!$conn) { die("Connection failed: " . mysqli_connect_error()); } ?>
index.php
This contains our login form but you can access a link here if you want to sign up.
<?php include('conn.php'); if(isset($_SESSION['id'])){ header('location:home.php'); } ?> <!DOCTYPE html> <html> <head> <title>Sign up Form with Email Verification in PHP/MySQLi</title> <script src="<a href="https://ajax.googleapis.com/ajax/libs/jquery/3.1.0/jquery.min.js"></script> " rel="nofollow">https://ajax.googleapis.com/ajax/libs/jquery/3.1.0/jquery.min.js"></scri...</a> <script src="<a href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/js/bootstrap.min.js"></script> " rel="nofollow">https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/js/bootstrap.min.js"></s...</a> <link href="<a href="https://fonts.googleapis.com/css?family=Open+San"> " rel="nofollow">https://fonts.googleapis.com/css?family=Open+San"> </a> <link href="<a href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css"> " rel="nofollow">https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min....</a> <link rel="stylesheet" href="<a href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css">" rel="nofollow">https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css"></a> <style> #login_form{ width:350px; position:relative; top:50px; margin: auto; padding: auto; } </style> </head> <body> <div class="container"> <div id="login_form" class="well"> <h2><center><span class="glyphicon glyphicon-lock"></span> Please Login</center></h2> <hr> <form method="POST" action="login.php"> Email: <input type="text" name="email" class="form-control" required> <div style="height: 10px;"></div> Password: <input type="password" name="password" class="form-control" required> <div style="height: 10px;"></div> <button type="submit" class="btn btn-primary"><span class="glyphicon glyphicon-log-in"></span> Login</button> No account? <a href="signup.php"> Sign up</a> </form> <div style="height: 15px;"></div> <?php if(isset($_SESSION['log_msg'])){ ?> <div style="height: 30px;"></div> <div class="alert alert-danger"> <span><center> <?php echo $_SESSION['log_msg']; unset($_SESSION['log_msg']); ?> </center></span> </div> <?php } ?> </div> </div> </body> </html>
signup.php
This is our sign up form.
<!DOCTYPE html> <html> <head> <title>Sign up Form with Email Verification in PHP/MySQLi</title> <script src="<a href="https://ajax.googleapis.com/ajax/libs/jquery/3.1.0/jquery.min.js"></script> " rel="nofollow">https://ajax.googleapis.com/ajax/libs/jquery/3.1.0/jquery.min.js"></scri...</a> <script src="<a href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/js/bootstrap.min.js"></script> " rel="nofollow">https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/js/bootstrap.min.js"></s...</a> <link href="<a href="https://fonts.googleapis.com/css?family=Open+San"> " rel="nofollow">https://fonts.googleapis.com/css?family=Open+San"> </a> <link href="<a href="https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min.css"> " rel="nofollow">https://maxcdn.bootstrapcdn.com/font-awesome/4.7.0/css/font-awesome.min....</a> <link rel="stylesheet" href="<a href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css"> <style> " rel="nofollow">https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css"> ...</a> #signup_form{ width:350px; position:relative; top:50px; margin: auto; padding: auto; } </style> </head> <body> <div class="container"> <div id="signup_form" class="well"> <h2><center><span class="glyphicon glyphicon-user"></span> Sign Up</center></h2> <hr> <form method="POST" action="register.php"> Email: <input type="text" name="email" class="form-control" required> <div style="height: 10px;"></div> Password: <input type="password" name="password" class="form-control" required> <div style="height: 10px;"></div> <button type="submit" class="btn btn-primary"><span class="glyphicon glyphicon-save"></span> Sign Up</button> <a href="index.php"> Back to Login</a> </form> <?php if(isset($_SESSION['sign_msg'])){ ?> <div style="height: 40px;"></div> <div class="alert alert-danger"> <span><center> <?php echo $_SESSION['sign_msg']; unset($_SESSION['sign_msg']); ?> </center></span> </div> <?php } ?> </div> </div> </body> </html>
register.php
This contains our signup code as well as our send verification email code.
<?php include('conn.php'); if ($_SERVER["REQUEST_METHOD"] == "POST") { function check_input($data){ $data=trim($data); $data=stripslashes($data); $data=htmlspecialchars($data); return $data; } $email=check_input($_POST['email']); $password=md5(check_input($_POST['password'])); if (!filter_var($email, FILTER_VALIDATE_EMAIL)) { $_SESSION['sign_msg'] = "Invalid email format"; header('location:signup.php'); } else{ $query=mysqli_query($conn,"select * from user where email='$email'"); if(mysqli_num_rows($query)>0){ $_SESSION['sign_msg'] = "Email already taken"; header('location:signup.php'); } else{ //depends on how you set your verification code $set='123456789abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ'; $code=substr(str_shuffle($set), 0, 12); mysqli_query($conn,"insert into user (email, password, code) values ('$email', '$password', '$code')"); $uid=mysqli_insert_id($conn); //default value for our verify is 0, means it is unverified //sending email verification $to = $email; $subject = "Sign Up Verification Code"; $message = " <html> <head> <title>Verification Code</title> </head> <body> <h2>Thank you for Registering.</h2> <p>Your Account:</p> <p>Email: ".$email."</p> <p>Password: ".$_POST['password']."</p> <p>Please click the link below to activate your account.</p> <h4><a href='<a href="http://localhost/send_mail/activate.php?uid=" rel="nofollow">http://localhost/send_mail/activate.php?uid=</a>$uid&code=$code'>Activate My Account</h4> </body> </html> "; //dont forget to include content-type on header if your sending html $headers = "MIME-Version: 1.0" . "\r\n"; $headers .= "Content-type:text/html;charset=UTF-8" . "\r\n"; $headers .= "From: <a href="mailto:webmaster@sourcecodester.com" rel="nofollow">webmaster@sourcecodester.com</a>". "\r\n" . "CC: <a href="mailto:ndevierte@gmail.com" rel="nofollow">ndevierte@gmail.com</a>"; mail($to,$subject,$message,$headers); $_SESSION['sign_msg'] = "Verification code sent to your email."; header('location:signup.php'); } } } ?>
activate.php
The goto page from registered email. This is our verification page.
<?php include('conn.php'); if(isset($_GET['code'])){ $user=$_GET['uid']; $code=$_GET['code']; $query=mysqli_query($conn,"select * from user where userid='$user'"); $row=mysqli_fetch_array($query); if($row['code']==$code){ //activate account mysqli_query($conn,"update user set verify='1' where userid='$user'"); ?> <p>Account Verified!</p> <p><a href="index.php">Login Now</a></p> <?php } else{ $_SESSION['sign_msg'] = "Something went wrong. Please sign up again."; header('location:signup.php'); } } else{ header('location:index.php'); } ?>
login.php
Our login code that will check if the user has successfully sign up and verified its account.
<?php include('conn.php'); if ($_SERVER["REQUEST_METHOD"] == "POST") { function check_input($data) { $data = trim($data); $data = stripslashes($data); $data = htmlspecialchars($data); return $data; } $email=check_input($_POST['email']); $password=md5(check_input($_POST['password'])); if (!filter_var($email, FILTER_VALIDATE_EMAIL)) { $_SESSION['log_msg'] = "Invalid email format"; header('location:index.php'); } else{ $query=mysqli_query($conn,"select * from user where email='$email' and password='$password'"); if(mysqli_num_rows($query)==0){ $_SESSION['log_msg'] = "User not found"; header('location:index.php'); } else{ $row=mysqli_fetch_array($query); if($row['verify']==0){ $_SESSION['log_msg'] = "User not verified. Please activate account"; header('location:index.php'); } else{ $_SESSION['id']=$row['userid']; header('location:index.php'); } } } } ?>
home.php
This page will show if the user that login is verified.
logout.php
Lastly, our logout that will destroy our login session.
<?php header('location:index.php'); ?>
That ends this tutorial. If you have any questions or comment, feel free to write it below or message me. Happy Coding :)