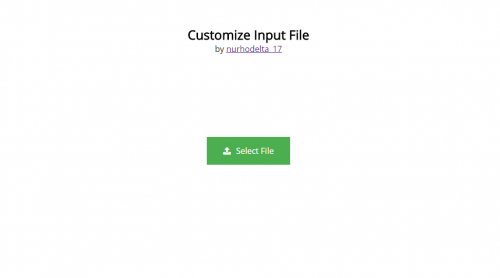
This tutorial will teach you on how to customize input file using jQuery and CSS.
Note: Scripts and css used in this tutorial are hosted, therefore, you need internet connection for them to work.
Hiding our Input File
First step is to hide our input file. It will look something like this.
<input type="file" name="file" id="file" style="display:none;" multiple>
Creating a Substitute Button
Next we create a substitute button and will set a jQuery function that whenever this button is clicked, our input file will be clicked. It will look something like this.
Creating our Example
Here's an example for you to further understand the topic.
index.html
We create our input file, substitute button, our css and source to our jQuery code in this page.
<!DOCTYPE html> <html> <head> <script src="<a href="https://ajax.googleapis.com/ajax/libs/jquery/3.1.0/jquery.min.js"></script> <style> body{ font-family: 'Open Sans', sans-serif; } .button { background-color: #4CAF50; border: none; color: white; padding: 16px 32px; text-align: center; text-decoration: none; font-size: 16px; margin: 4px 2px; cursor:pointer; font-family: 'Open Sans', sans-serif; } .hidden{ display:none; } .h10{ height:10px; } #filetext{ margin-left:10px; } .title{ font-size:25px; } .container { margin : 50px auto 0 auto; width:50%; } #filebutton{ margin : 150px auto 0 auto; } </style> </head> <body> <div id="container"> <div class="container"> <input type="file" name="file" id="file" class="hidden" multiple> </div> </div> </body> </html>
custom.js
Lastly, our jQuery code.
$(document).ready(function(){ $('#filebutton').click(function(){ $('#file').click(); }); $('#file').change(function(){ var name = $(this).val().split('\\').pop(); var file = $(this)[0].files; if(name != ''){ if(file.length >=2){ $('#filetext').html(file.length + ' files ready to upload'); } else{ $('#filetext').html(name); } } }); });
That ends this tutorial. If you have any comments or questions, feel free to write it below or message me. Happy Coding :)