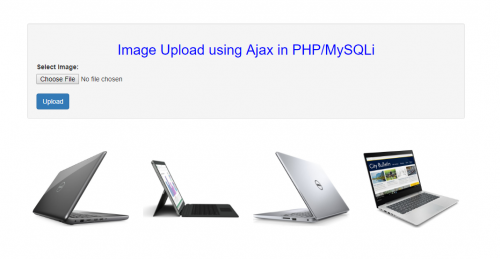
Creating our Database
First step is to create our database.
1. Open phpMyAdmin.
2. Click databases, create a database and name it as upload.
3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction.
1. Open phpMyAdmin.
2. Click databases, create a database and name it as upload.
3. After creating a database, click the SQL and paste the below codes. See image below for detailed instruction.
CREATE TABLE `photo` ( `photoid` INT(11) NOT NULL AUTO_INCREMENT, `location` VARCHAR(150) NOT NULL, PRIMARY KEY(`photoid`) ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
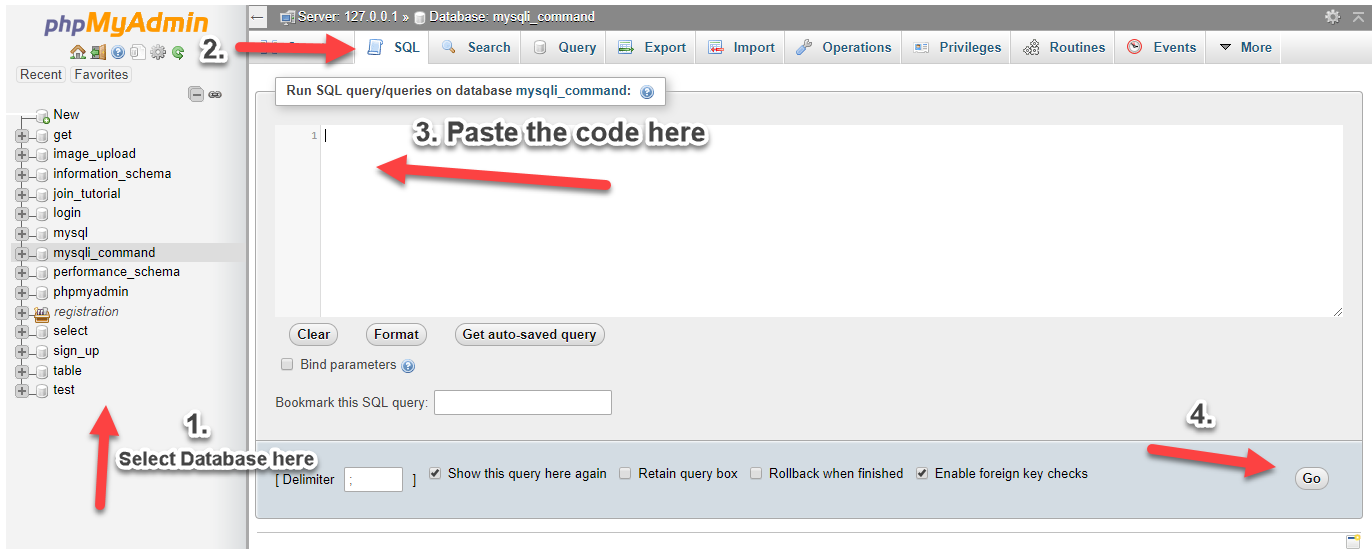
Creating our Connection
Next, we create our connection to our database. This will serve as the bridge between our forms and database. We name this as conn.php.
<?php if (!$conn) { } ?>
index.php
We create our upload form and we show the images uploaded in this page.
<!DOCTYPE html> <html> <head> <title>Image Upload using AJAX in PHP/MySQLi</title> <script src="<a href="https://ajax.googleapis.com/ajax/libs/jquery/3.1.0/jquery.min.js"></script> " rel="nofollow">https://ajax.googleapis.com/ajax/libs/jquery/3.1.0/jquery.min.js"></scri...</a> <link rel="stylesheet" href="<a href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css"" rel="nofollow">https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css"</a> /> <script src="<a href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script> </head> <body> <div" rel="nofollow">https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></s...</a> class="container"> <div style="height:50px;"></div> <div class="row"> <div class="well" style="width:80%; padding:auto; margin:auto"> <form> <h2 align="center" style="color:blue">Image Upload using AJAX in PHP/MySQLi</h2> <label>Select Image:</label> <input type="file" name="file" id="file"><br> <button type="button" id="upload_button" class="btn btn-primary">Upload</button> </form> </div> </div> <div style="height:50px;"></div> <div style="width:80%; padding:auto; margin:auto;"> <div id="image_area"></div> </div> </div> </body> <script src="custom.js"></script> </html>
fetch_photo.php
This is our code in fetching uploaded photos from our database.
<?php include('conn.php'); $inc=4; $inc = ($inc == 4) ? 1 : $inc+1; if($inc == 1) echo '<div class="row">'; ?> <div class="col-lg-3"><img src="<?php echo $row['location']?>" style="height:200px; width:100%;"></div> <?php if($inc == 4) echo '</div>'; } if($inc == 1) echo '<div class="col-lg-3"></div><div class="col-lg-3"></div><div class="col-lg-3"></div></div>'; if($inc == 2) echo '<div class="col-lg-3"></div><div class="col-lg-3"></div></div>'; if($inc == 3) echo '<div class="col-lg-3"></div></div>'; } ?>
upload.php
This is our code in uploading images into our database.
<?php include('conn.php'); if($_FILES["file"]["name"] != '') { $location = 'upload/' . $newFilename; } ?>
custom.js
Lastly, this contains our jQuery and AJAX code in uploading and fetching our images.
$(document).ready(function(){ showPhoto(); $(document).on('click', '#upload_button', function(){ var name = document.getElementById("file").files[0].name; var form_data = new FormData(); var ext = name.split('.').pop().toLowerCase(); if(jQuery.inArray(ext, ['gif','png','jpg','jpeg']) == -1){ alert("Invalid Image File"); } var oFReader = new FileReader(); oFReader.readAsDataURL(document.getElementById("file").files[0]); var f = document.getElementById("file").files[0]; var fsize = f.size||f.fileSize; if(fsize > 2000000){ alert("Image File Size is very big"); } else{ form_data.append("file", document.getElementById('file').files[0]); $.ajax({ url:"upload.php", method:"POST", data: form_data, contentType: false, cache: false, processData: false, success:function(){ showPhoto(); } }); } }); }); function showPhoto(){ $.ajax({ url:"fetch_photo.php", method:"POST", data:{ fetch:1, }, success:function(data){ $('#image_area').html(data); } }); }
That ends this tutorial. If you have any comments or questions, feel free to comment below or message me. Happy Coding :)