This tutorial will give you an idea on how to use the stored cookie to login and I've added a "logout" function that destroys both session and cookie.
Creating our Database
First, we're going to create a database that contains our data.
1. Open phpMyAdmin.
2. Click databases, create a database and name it as "cookie".
3. After creating a database, click the SQL and paste the below code. See image below for detailed instruction.
1. Open phpMyAdmin.
2. Click databases, create a database and name it as "cookie".
3. After creating a database, click the SQL and paste the below code. See image below for detailed instruction.
CREATE TABLE `user` ( `userid` INT(11) NOT NULL AUTO_INCREMENT, `username` VARCHAR(30) NOT NULL, `password` VARCHAR(30) NOT NULL, `fullname` VARCHAR(60) NOT NULL, PRIMARY KEY (`userid`) ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
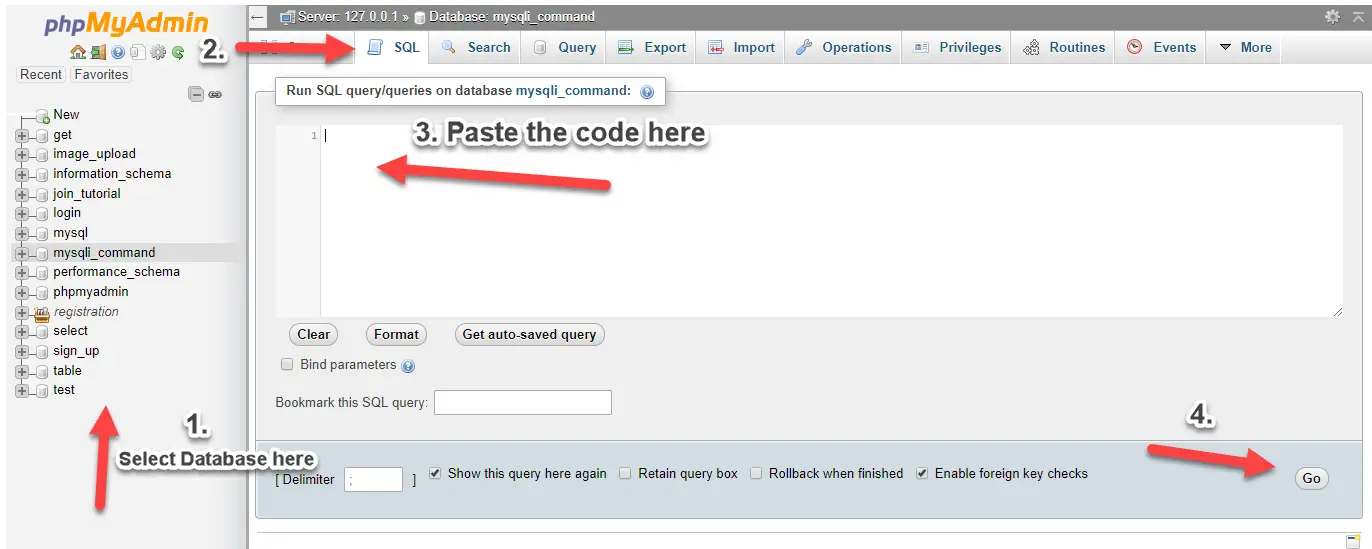
Inserting Data into our Database
Next, we insert users into our database. This will be our reference when we login.
1. Click the database the we created earlier.
2. Click SQL and paste the code below.
1. Click the database the we created earlier.
2. Click SQL and paste the code below.
INSERT INTO `user` (`username`, `password`, `fullname`) VALUES ('neovic', 'devierte', 'neovic devierte'), ('lee', 'ann', 'lee ann');
Creating our Connection
Next step is to create a database connection and save it as "conn.php". This file will serve as our bridge between our form and our database. To create the file, open your HTML code editor and paste the code below after the tag.
<?php // Check connection { } ?>
Creating our Login Form
Next is to create our login form. In this form, I've added a code that if ever there's a cookie stored, it will show in the login inputs. To create the form, open your HTML code editor and paste the code below after the tag. We name this as "index.php".
<?php include('conn.php'); ?> <!DOCTYPE html> <html> <head> <title>Login Using Cookie with Logout</title> </head> <body> <h2>Login Form</h2> <form method="POST" action="login.php"> <label>Username:</label> <input type="text" value="<?php if (isset($_COOKIE["user"])){echo $_COOKIE["user"];}?>" name="username"> <label>Password:</label> <input type="password" value="<?php if (isset($_COOKIE["pass"])){echo $_COOKIE["pass"];}?>" name="password"><br><br> <input type="checkbox" name="remember"> Remember me <br><br> <input type="submit" value="Login" name="login"> </form> <span> <?php echo $_SESSION['message']; } ?> </span> </body> </html>
Creating our Login Script
Next step is creating our login script. We name this script as "login.php".
<?php include('conn.php'); $username=$_POST['username']; $password=$_POST['password']; $query=mysqli_query($conn,"select * from `user` where username='$username' && password='$password'"); $_SESSION['message']="Login Failed. User not Found!"; } else{ //set up cookie } $_SESSION['id']=$row['userid']; } } else{ $_SESSION['message']="Please Login!"; } ?>
Creating our Login Success Page
Next, we create a go to page if login is successful. This page will show the user that login successfully. We name this page as "success.php". In this page, I've added the logout link to destroy both session and cookie.
<?php } include('conn.php'); ?> <!DOCTYPE html> <html> <head> <title>Setting Up Cookie on User Login</title> </head> <body> <h2>Login Success</h2> <?php echo $row['fullname']; ?> <br> <a href="logout.php">Logout</a> </body> </html>
Creating our Logout Script
Last is our logout script. This script destroys both our session and cookie then redirect us back to our login page. We name the script as "logout.php".
<?php } ?>